Tool Script module <PRO>
This Lua module is used in the tool editor and provides functions to create the tool geometry. This module can be used to create construction elements such as lines and arcs, from which the rotation profile for the tool is generated. The orientation and dimensions of the construction elements are subject to certain restrictions:
- The elements must not cross the tool axis
- The elements must not create an undercut in both directions in the geometry
In other words, the end point of an element always has a larger value or the same value in one direction than the start point.
Script sequence
A tool script works according to the following principle: the script is processed from the top line to the last line. Before the command for the creation of an element is given, the parameters for this element are defined by functions. After creating an element, all parameters are reset and must be set again for the following element.
The tool is designed starting from the tool nose. The first element always starts at X0 and Z0. The geometry always ends at the maximum radius of the tool end, usually at the shank end.
The following example script for the generation of a Bull End Mill will illustrate this:
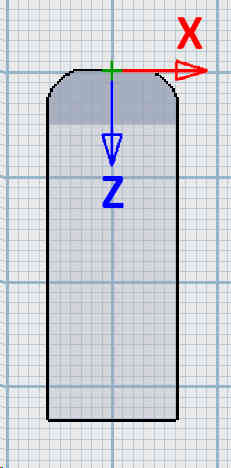
to_x(3) -- The next element
-- ends at position X3
to_z(0) -- The next element
-- ends at position Z0
line() -- Create a line with
-- the set parameters
tangent() -- The next one joins the
-- last element tangentially
arc_rad(3) -- The arc radius is 3
slope_out(0) -- The exit angle is
-- parallel to the tool axis
arc_a() -- Create an arc in the
-- clockwise
tangent() -- The next one joins the
-- last element tangentially
length(30) -- and the line has a
-- length of 30
line() -- create the line with the
-- set parameters
cutedge(5) -- The cutting edge of the tool
-- reaches up to Z5
Parameter functions
All lengths are interpreted as user units.
Not all functions are compatible with each other, since some combinations can create contradictions. For example, it is not possible to specify an angle with slope_in() if the target point of a line has already been determined by to_x() and to_z() and the angle is derived from it.
Another example concerns an arc that is tangent to a line using the tangent() function. In this case, the angle of entry is already specified and cannot be additionally specified with slope_in().
Function | slope_in(grad) |
---|---|
Element | Arcs, lines |
Description | Specifies the entry angle for the next element. |
Value range | 0°-90° 0°: The angle is parallel to the tool axis (vertical) 90°: The angle is horizontal |
Example | slope_in(45) -- eg. for engraving tool tip |
Function | slope_out(grad) |
---|---|
Element | Bögen |
Description | Specifies the exit angle for the arc. |
Value range | 0°-90° 0°: The angle is parallel to the tool axis (vertical) 90°: The angle is horizontal |
Example | slope_out(0) -- Element ends in vertical alignment |
Function | tangent() |
---|---|
Element | Arcs, lines |
Description | The next element joins the last element tangentially. This function determines the entry radius of the next element. |
Example | tangent() -- Element connects tangentially. |
Function | to_x(abs_position) |
---|---|
Element | Lines |
Description | Specifies the absolute X target position of the line. The line does not necessarily have to run horizontally.. |
Value range | The target position must be greater than or equal to the start position. |
Example | to_x(30) -- Line ends at position X30 |
Function | to_z(abs_position) |
---|---|
Element | Lines |
Description | Specifies the absolute Z target position of the line. The line does not necessarily have to be vertical. |
Value range | The target position must be greater than or equal to the start position. |
Example | to_x(30) -- Line ends at position X30 |
Function | length(wert) |
---|---|
Element | Lines |
Description | Determines the length of the line, regardless of the orientation |
Value range | > 0 |
Example | length(5) -- The line has a length of 5 |
Function | arc_rad(radius) |
---|---|
Element | Arcs |
Description | Determines the radius for an arc. |
Value range | > 0 |
Example | arc_rad(3) -- The arc has a radius of 3 |
Generation
The following functions create the design elements based on the previously defined parameters.
Optionally, an error description string can be passed as an argument. If the element creation fails, this error description will be displayed as an error message. This facilitates the localization of errors in complex geometries.
Function | line(optional_error_string) |
---|---|
Element | Line |
Description | Creates a line with the previously defined parameters. It is necessary to ensure that the value for the end point is greater than or equal to the start point in both directions. |
Example | line("Shaft transition could not be created.") |
Function | arc_a(optional_error_string) |
---|---|
Element | Clockwise arc |
Description | Creates a clockwise arc with the previously defined parameters. Regardless of the calculation of the entry and exit angles, it must be ensured that the exit angle is smaller than the entry angle. |
Example | arc_a("Arc for tool nose could not be created") |
Function | arc_b(optional_error_string) |
---|---|
Element | Arc counterclockwise |
Description | Creates an arc counterclockwise with the previously defined parameters. Regardless of the calculation of the entry and exit angles, it must be ensured that the exit angle is greater than the entry angle. |
Example | arc_b("Arc for Roundover could not be created") |
General functions
The following functions concern the tool in general.
Function | cutedge(position_z) |
---|---|
Description | The cutting edge of the tool extends to this height. The function is necessary for all tools. It is independent of other functions and can be located anywhere in the script. |
Value range | > 0 |
Example | cutedge(12) -- The tool cutting geometry ranges up to Z12 |
Function | autocolltol() |
---|---|
Description | This command activates the automatic calculation of the collision tolerance. The collision tolerance is described in more detail in the chapter about predefined tools. |
Example | autocolltol() -- Activates the automatic calculation of the collision tolerance |